This is a article that grew out of a answer I was writing for a question that I was asked in the thread, What programming language do you know?
As I was writting it, more people asked me similar questions (Including PM) and after realising there are people who want to get started on programming, I thought I would expand it into an article and dedicate its own thread to it.
I was initially addressing one person, so pardon me for the way its written.
Its very hard to tell what "the important language" for you is without an end in mind. For solid foundations of programming (since that is your stated interest) then I would say to focus on a language-agnostic approach, as in dont focus on language specific features but on learning solid principles of programming, this is what counts long term anyways.
Of course, on top of having to learn a bunch of names and concepts, you dont want to further add to the difficulties by doing your exercises and programming in "machine code" (an arrangement of "0" and "1") or something else around that end of the deep:
Python is at the shallow end of that same deep, and yet you would not be incurring a noticeable trade-off for picking it, why is it at the shallow end? Its the closest language to "plain English", on top of that:
Remember, dont use python to just learn python, use it as a driver to focus on core programming concepts.
And of course the following which is found in most languages:
Start with his first series, he is very comprehensive so he covers the above and minds good programming practices along the way:
Dont neglect the documentations, a video is more interesting than dry documentation but you risk in ending up with incomplete understanding of matters.
The people who wrote the documentation are the same people behind the designs and implementations of Python (or "X" thing), so as well as giving you insight into the why, they also provide a comprehensive A to Z explanation that is always up to date.
A good teacher of mine used to always tell us:
And my observation of my peers has borne it out, the pros always the docs open. Right now, I have three documentation tabs pinned (not that I am a pro):
Dont be hard on yourself if things are not sticking, that's what the docs are for, keep revisiting the relevant pages and manually typing and tinkering.
Its hard enough internalising all this, learning to appropriately name things (which is said to be the hardest thing in programming), imagine on top of that having to fight your tools.
Also Programmers are very fussy about their workstation, workflows and tools, there is no "right tool", its all personal and over time you will discover this yourself. Right now you need:
If you do the above, others will see you care about their time and will likely help you. Over time you will discover which communities tend to yield better insights and answers and you will naturally rank them accordingly.
For Python, I have discovered the following order be the most useful to me:
Feel free to cross post as well, sometimes you will get a nothing burger answer on website X, so if you take care to ask across two to three websites, you will likely wake up to more answers the following morning.
As I was writting it, more people asked me similar questions (Including PM) and after realising there are people who want to get started on programming, I thought I would expand it into an article and dedicate its own thread to it.
I was initially addressing one person, so pardon me for the way its written.
I will first answer the difficulty question, It largely depends on the field, Some fields are relatively easy and have a low entry barrier (web development), from then on, the difficulty scales relatively to how deep you are going in.You don't have permission to view the spoiler content. Log in or register now.
Its very hard to tell what "the important language" for you is without an end in mind. For solid foundations of programming (since that is your stated interest) then I would say to focus on a language-agnostic approach, as in dont focus on language specific features but on learning solid principles of programming, this is what counts long term anyways.
Of course, on top of having to learn a bunch of names and concepts, you dont want to further add to the difficulties by doing your exercises and programming in "machine code" (an arrangement of "0" and "1") or something else around that end of the deep:
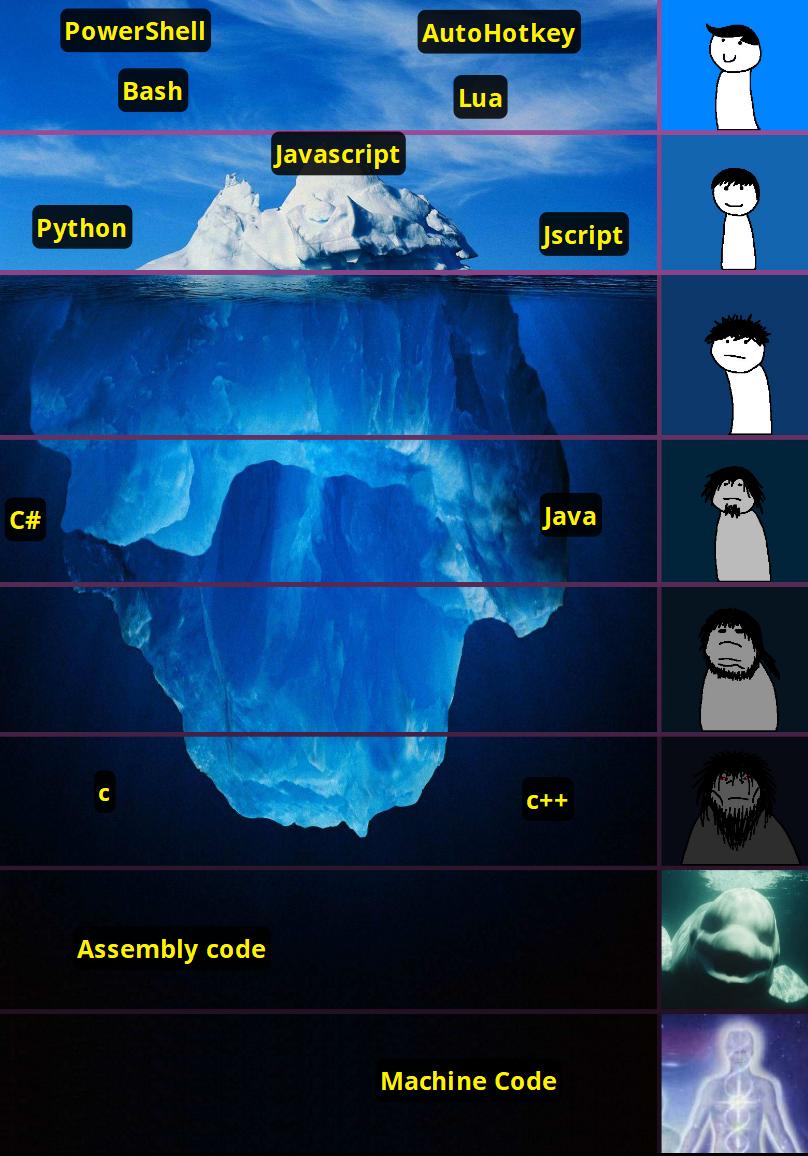
Python is at the shallow end of that same deep, and yet you would not be incurring a noticeable trade-off for picking it, why is it at the shallow end? Its the closest language to "plain English", on top of that:
- It is mature (30+ years old) so just about everything is figured out and settled
- Its second selling point which is "Batteries included", its standard library is extensive (add more features, as you need them)
- It has the largest community, so you will not be short of high quality learning material nor QnA forums to reach out to (when you are stuck)
- Has a wide use in open source projects (After a certain point, growth is only obtained through collaboration)
Remember, dont use python to just learn python, use it as a driver to focus on core programming concepts.
What is "core programming concepts" anyways?
A core part of programming, as I tried to explain in my other post is not understanding syntax (grammar) and semantics but how to approach a task/project and when you find yourself in a pit inside of a pit, how to break down the problem, and then further break it down, this is known as decomposition/compositionAnd of course the following which is found in most languages:
- Good naming conventions, documenting and maintaining code
- Variables and their types
- Operators
- Conditionals
- Loops
- Functions/methods (with the _call stack_)
- Arrays (Lists in Python)
- Regular Expressions
- Encapsulation
- Program flow
- File I/O
Start with his first series, he is very comprehensive so he covers the above and minds good programming practices along the way:
- Python 3: Fundamentals (40+ hours long)
- Python 3: Deep Dive (Part 1 - Functional)
- Python 3: Deep Dive (Part 2 - Iterators, Generators)
- Python 3: Deep Dive (Part 3 - Dictionaries, Sets, JSON)
- Python 3: Deep Dive (Part 4 - OOP)
"The pros always have the docs open"
Other than the above course I recommended, you should not need anything else other than the documentation for python, which is at https://docs.python.org/3/.Dont neglect the documentations, a video is more interesting than dry documentation but you risk in ending up with incomplete understanding of matters.
The people who wrote the documentation are the same people behind the designs and implementations of Python (or "X" thing), so as well as giving you insight into the why, they also provide a comprehensive A to Z explanation that is always up to date.
A good teacher of mine used to always tell us:
>The difference between the pros and the noobs is that the pros always have the documentation open
And my observation of my peers has borne it out, the pros always the docs open. Right now, I have three documentation tabs pinned (not that I am a pro):
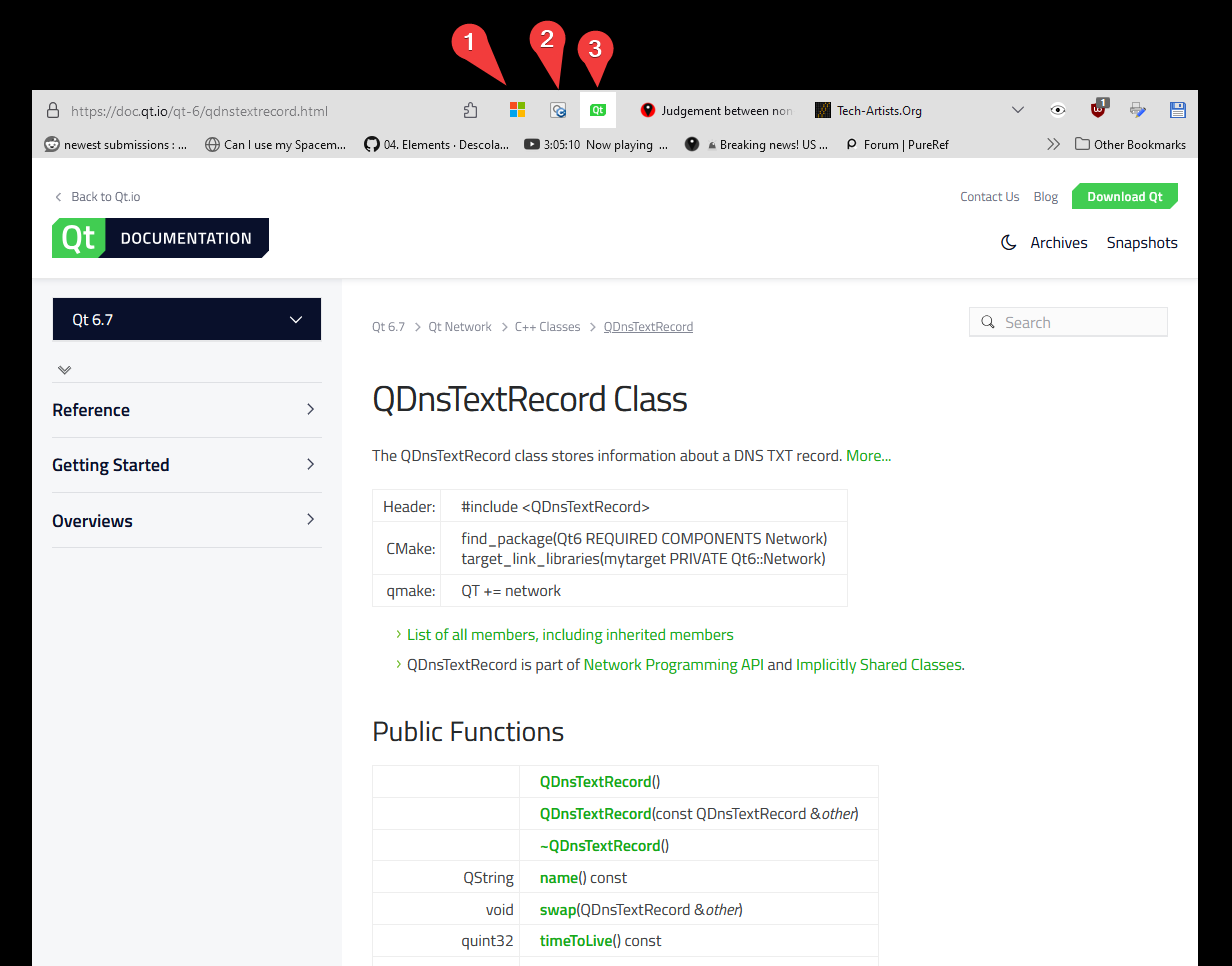
Dont be hard on yourself if things are not sticking, that's what the docs are for, keep revisiting the relevant pages and manually typing and tinkering.
Quality of life tools
Dont leave yourself open to a "death by a thousand paper cuts", invest in your tools and you will have a smooth experience. If something is not right or broken fix it, dont let it break you flow and tolerate it because its a "small thing", it add up and it will make your work not be pleasurable, and before you know it, you are avoiding it.Its hard enough internalising all this, learning to appropriately name things (which is said to be the hardest thing in programming), imagine on top of that having to fight your tools.
Also Programmers are very fussy about their workstation, workflows and tools, there is no "right tool", its all personal and over time you will discover this yourself. Right now you need:
- A source code editor - Just go with Visual Studio Code, its the most comprehensive option
- A documentation tool, Visual Studio Code also doubles as a Markdown editor (for formatting your text)
- A good video player, these days majority of your learning will be video. A 40 hour course sped by 2x could be a 20 hour course. (you get used to the speed)
- A shell (command line) for doing generic tasks and running build tools, use PowerShell, its already on your system and its versatile.
- A terminal, which is used to host your shell and interact with it. Windows Terminal is already on your system and its versatile.
Getting effective help
Its inevitable that you will get stuck, the good news is these days, its very easy to get help and people are more than glad to help someone who is trying in earnest, however no one likes someone that just wants his work done for him, to spare you any calacaal from being ignored or slighted do the following.- Make sure your question is on topic, that you are asking a suitable community
- The title or question should be clear, and summarises your post
- Describe what you are trying to do in the first place (imagine you are talking to someone else)
- Describe what is stopping you or breaking you code, so that others can recreate it on their end in order to diagnose it
- Include any errors or bugs you are dealing with in the post, dont take pictures of it, copy and paste it in.
If you do the above, others will see you care about their time and will likely help you. Over time you will discover which communities tend to yield better insights and answers and you will naturally rank them accordingly.
For Python, I have discovered the following order be the most useful to me:
Feel free to cross post as well, sometimes you will get a nothing burger answer on website X, so if you take care to ask across two to three websites, you will likely wake up to more answers the following morning.
Last edited: